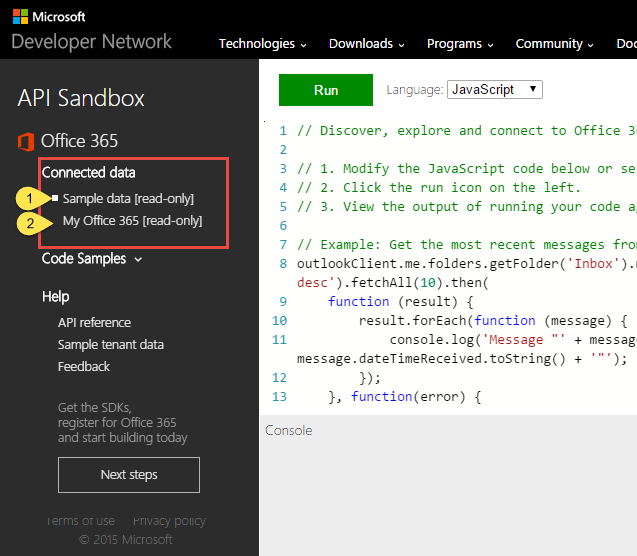
Office 365 API Sandbox - Learn how to use the API's with your own tenant data!
Post updated 2015-02-25 to include support for C#
Up until recently I’ve been using a lot of browser extensions and client-side tools for running test-queries and sample-code toward real data or developer-data in our tenants using the REST API in Office 365.
While tools like Advanced REST for Chrome are awesome, Microsoft has recently released the “API Sandbox” for Office 365 which essentially gives you a way to directly write code in the browser and test it – either using sample data or using your real tenant.
Getting started with the API Sandbox
First, you’ll head on to https://apisandbox.msdn.microsoft.com and you’ll see this interface where you have a menu on the left and a code-area to the right. You’ll see that you have two options for what data you want to connect to your query.
- Sample Data
- My Office 365
The Sample Data option gives you some demo-data provided by the Office 365 API Sandbox, which is essentially just a basic data structure provided to aid you in designing proper queries. It works very well, but may not always contain the exact details of your own tenants, which brings us to point number two.
The My Office 365 option enables you to authenticate to your own Office 365 tenants and make sure the API Sandbox is targeting your own production, test or development environments.
Run some sample code
Upon loading the API Sandbox, there’s a sample-snippet with JavaScript which allows you to just click a button and test the query instantaneously. Here’s what the sample can look like:
// Discover, explore and connect to Office 365 APIs
// 1. Modify the JavaScript code below or select a sample.
// 2. Click the run icon on the left.
// 3. View the output of running your code against a sample Office 365 tenant.
// Example: Get the most recent messages from a user's inbox
outlookClient.me.folders.getFolder('Inbox').messages.getMessages().orderBy('DateTimeReceived desc').fetchAll(10).then(
function (result) {
result.forEach(function (message) {
console.log('Message "' + message.subject + '" received at "' + message.dateTimeReceived.toString() + '"');
});
}, function(error) {
console.log(error);
}
);
If you hit “Run” it will populate the “Console” area with the results of your query. In the following image I’m using the built-in samples and without any modification I’m just hitting run – the results are displayed and the query seems to work.
Hey, I want to use my own Office 365 tenant data!
Right you are – its always more fun to play with live data whether it be production, test or development environments. Static sample-data doesn’t always give us what we want. So, the next step is of course to authenticate to your Office 365 tenant and enable you to run queries on your own data instead!
Authenticate to your tenant
By clicking the “My Office 365 [read-only]” link in the left navigation, you’ll bring up an authentication dialog where you’ll have to authenticate to your tenant.
Once you have entered your credentials and authenticated, you can see that you’re logged in to your tenant in the API Sandbox:
Now you can query your own data
If you specify some code to fetch information from your environment, you’ll see that the result that is generated will be your own data!
Actually, if you just want to run the same code as you did in the sample data, you just hit “Run” again and you’ll see that the results from that query will display your own data instead!
Why is this a good thing?
This is cool because not only does the API Sandbox allow me to test my production-ready queries against my actual data quickly, it also helps me with testing sample snippets and code from around the web in order to ensure they will do what I want them to do.
So, it’s all good?
Well, the tool is still in a preview so I guess there’s a lot more things to come. My experience is that its slower to use this tool than it is to use the Chrome extensions for debugging REST queries, but I’m hopeful that it will speed up once it comes out of preview.
I want to try other languages too!
Update 2015-02-25: C# now also supported!
As of the latest update to the API Sandbox, both C# and JavaScript are now supported.
JavaScript is currently the supported language to perform these queries with.
There are UserVoice votes going on to make sure more languages are included, should you want to use something other than the currently existing languages.
In the upper right corner of the editing area, you can see the available languages and also a dropdown to select more languages:
Should you select any of the currently unsupported languages, it will bring you to a dialog which is linked to the Visual Studio UserVoice site where you can vote for new features and languages in the API Sandbox:
Tests directly from MSDN
Another really cool thing that Microsoft has done right is the modern MSDN documentation for the Office 365 REST API’s.
If you check out the API reference here and select one of the API documents, for example the “List folder contents” under the Files API:
You’ll see a long document with a lot of information about various tasks and queries you can perform through the API.
Let’s say I’m interested in testing the Files API to get all the contents of a specific folder in my OneDrive for Business (the “Shared with Everyone” folder in this case), you can see below that there’s a really intuitive and easy way to test all the code samples directly from the MSDN documentation (awesome!).
- Sample REST query that will be performed using GET.
- If you want to modify the input variable to the query (which folder to query in this case) you can do that directly from the MSDN page before clicking Try.
- Click this magic button and it will automatically execute your request and give you a sample response.
- The response is JSON and well-formatted
Full sample response from the sample query can look like this:
{
"@odata.context": "https://a830edad9050849NDA1-my.sharepoint.com/_api/v1.0/$metadata#files",
"value": [
{
"@odata.type": "#Microsoft.FileServices.File",
"@odata.id": "https://a830edad9050849NDA1-my.sharepoint.com/_api/v1.0/files/01J5374RUS7SZXIQXTTBGYQ2BAOKATKQFQ",
"@odata.etag": "\"{74B3FC92-F342-4D98-8868-2072813540B0},1\"",
"@odata.editLink": "files/01J5374RUS7SZXIQXTTBGYQ2BAOKATKQFQ",
"createdBy": {
"application": null,
"user": {
"id": null,
"displayName": "Alex D"
}
},
"eTag": "\"{74B3FC92-F342-4D98-8868-2072813540B0},1\"",
"id": "01J5374RUS7SZXIQXTTBGYQ2BAOKATKQFQ",
"lastModifiedBy": {
"application": null,
"user": {
"id": null,
"displayName": "Alex D"
}
},
"name": "Denver Data.xlsx",
"parentReference": {
"driveId": "01OTIWNQQOTEPNCMVR3ZBZE7ZJTAODCBSU",
"id": "01J5374RTJSQOEUWQDHNHY7H5Y47ILXBPM",
"path": "/Shared with Everyone"
},
"size": 23373,
"dateTimeCreated": "2014-10-22T12:04:20Z",
"dateTimeLastModified": "2014-10-22T12:04:20Z",
"type": "File",
"webUrl": "https://a830edad9050849NDA1-my.sharepoint.com/alexd_a830edad9050849nda1_onmicrosoft_com/Documents/Shared%20with%20Everyone/Denver%20Data.xlsx",
"contentUrl": "https://a830edad9050849NDA1-my.sharepoint.com/alexd_a830edad9050849nda1_onmicrosoft_com/_api/v1.0/files/01J5374RUS7SZXIQXTTBGYQ2BAOKATKQFQ/content"
}
]
}
Summary
While the sandbox for trying out some code is awesome, there’s still a long way to go before being “the ultimate tool”. The main points that needs to be addressed are:
- Performance. Make this thing a lot faster and I would use it more often.
- Language availability. Make more languages (specifically C#) available through the API Sandbox.
(As of the latest update to include C# this point is no longer valid) - Visual Studio integration. Okay, perhaps this one isn’t necessary, but I would see some interesting applications for this!
Enjoy your new sandbox.
Recent comments