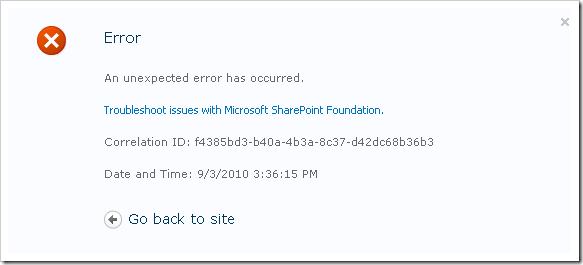
Find error messages with a Correlation ID token in SharePoint 2010
In SharePoint 2010, you’ve got some new capabilities for error reporting and logs. One of the most noted features for me as a developer is that whenever you bump into an error message – you’ll be presented with a correlation ID token.
In this article I will try to explain how you can fetch that token from SharePoint, and also provide a small snippet of code to be able to build your own “Log Searcher Web Part” for your administrators.
What is a Correlation ID in SharePoint?
In SharePoint 2010, you get a Correlation ID (which is a GUID) attached to your logs/error messages when something happens. This ID can then be used to lookup that specific error from the logs.
This Correlation ID is used per request-session in SharePoint 2010, and if you are in the process of requesting some information from SharePoint and bump into some problems along the way – your Correlation ID will be the best starting point for searching for what went wrong along that request!
You might get an error message like this in SharePoint 2010 (don’t worry, if you haven’t seen one like this one yet – just hang in there, sooner or later you will ;-)
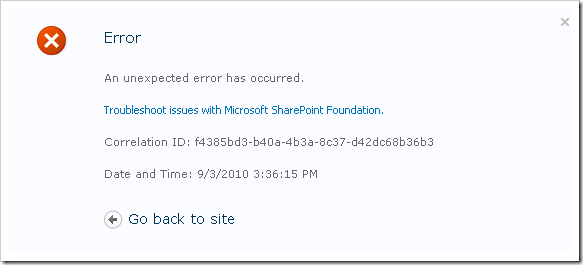
Search for the log messages based on the Correlation ID token
So if you’ve gotten such an error message and want to find out more information about the actual error and don’t have the time to go around poking in the logs and searching – there’s a few methods you can do this rather easily, as described below.
Using PowerShell to lookup a log message based on Correlation ID token
One of the quick-n-awesome ways to do this is to simply hook up a PowerShell console (SharePoint 2010 Management Shell) and then just write the following command (replace the with the Correlation Id):
get-splogevent | ?{$_.Correlation -eq "GUID" }
This will give you the details about your specific error like this in the console:
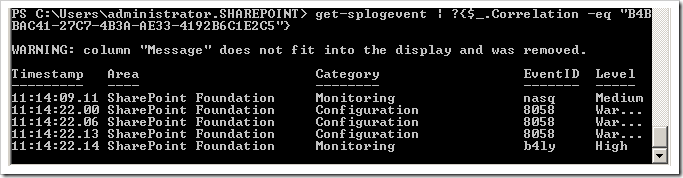
You might want to be more precise and get more specific details out of your query, then you can try something like this:
get-splogevent | ?{$_.Correlation -eq "GUID"} | select Area, Category, Level, EventID, Message | Format-List
This will give you the details about your specific error like this with some juicy details:
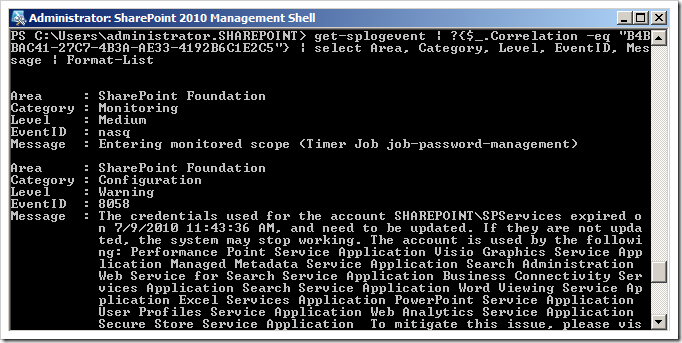
Finally if you would want these messages to be put into a text or log file instead, you could just add the classic “> C:Awesome.log” after the command like this:
get-splogevent | ?{$_.Correlation -eq "GUID"} | select Area, Category, Level, EventID, Message | Format-List > "C:\Awesome.log"
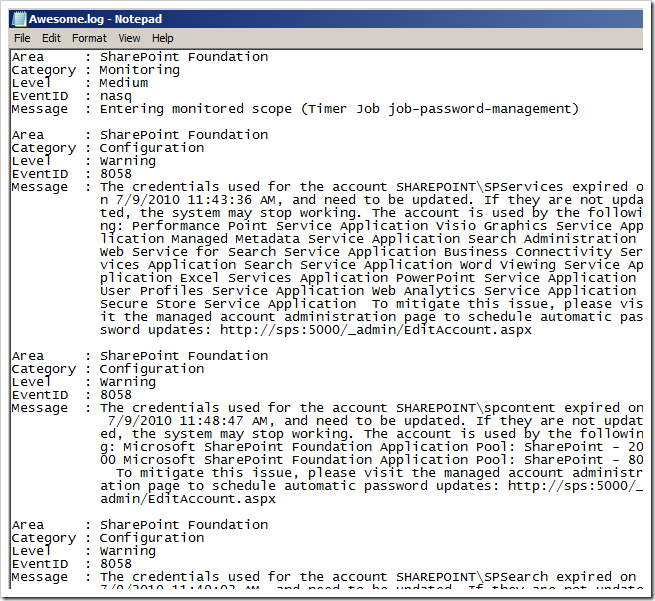
On MSDN they have an overvoew of using SP-GetLogEvent which I would recommend!
Using SQL queries to find log message based on Correlation ID token
You can utilize the SQL logs database to fetch the specific error message based on Correlation id as well. In your logging DB (usually called WSS\Logging
, but can be called something else if you’ve changed it) there is a view called ULSTraceLog
which you can query with a simple SQL query and fetch the results.
I’ve created a query to fetch the items from the logging database like this:
select [RowCreatedTime],[ProcessName],[Area],[Category],EventID,[Message]
from [WSS_UsageApplication].[dbo].[ULSTraceLog]
where CorrelationId='B4BBAC41-27C7-4B3A-AE33-4192B6C1E2C5'
This will render your results in the SQL Query window like this:
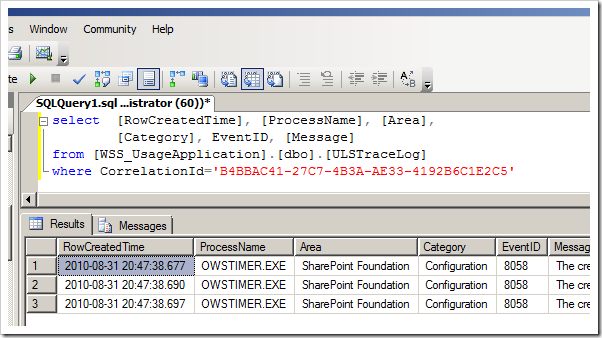
This can of course be implemented in a Web Part for your convenience as well (I’ve created a bunch of diag. and logging web parts that I’ve got deployed to Central Admin) that could look like this (this way you don’t need physical access to the ULS logs all the time, but can do a quick lookup from the web part):
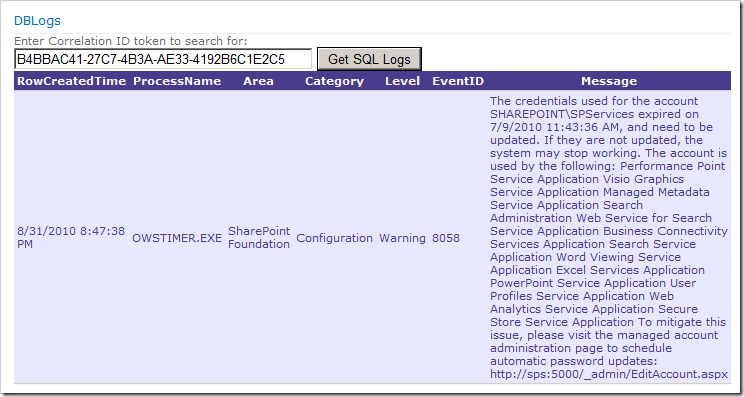
Get the current Correlation ID by using code
I got this piece of code from my good friend Wictor’s blog post. Thanks Wictor – this made my Logging-project more satisfying!
With the following code you can fetch the current Correlation Id of a request.
Create a method (in my case called GetCurrentCorrelationToken()) to wrap up the functionality of returning the current token like this:
public class CorrelationId
{
[DllImport ("advapi32.dll")]
public static extern uint EventActivityIdControl(uint controlCode,refGuid activityId);
publicconstuint EVENT_ACTIVITY_CTRL_GET_ID = 1;
publicstaticGuid GetCurrentCorrelationToken()
{
Guid g = Guid .Empty;
EventActivityIdControl(EVENT_ACTIVITY_CTRL_GET_ID, ref g);
return g;
}
}
Then from wherever in your code you can simply call it by using this approach:
protected void Button1_Click(object sender, EventArgs e)
{
Label1.Text = CorrelationId.GetCurrentCorrelationToken().ToString();
}
(Normally you might want this in a try/catch statement or something like that)
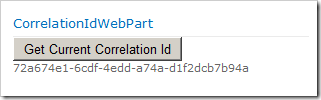
Summary
This article should give you (administrator and developer) an insight in how you easily can track the specific errors your users encounter in their/your application(s). If a user gets an error message in SharePoint 2010, they’ll also see the Correlation ID.
If you can train your users to write down that Correlation ID along with a few simple steps on how the error might have been occurring, you’re going to have a much easier way to find the details about that specific error than ever before.
Enjoy!
Recent comments