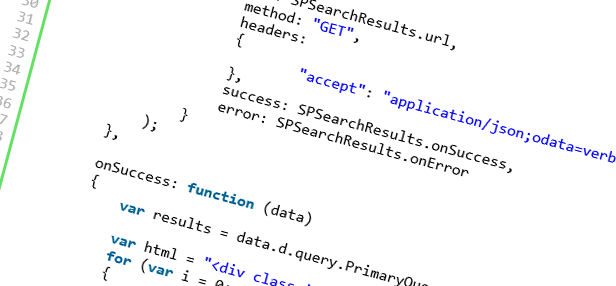
SP 2013: Searching in SharePoint 2013 using the REST new API's
Author: Tobias Zimmergren
http://www.zimmergren.net | http://www.tozit.com | @zimmergren
Introduction
Search has always been a great way to create custom solutions that aggregate and finds information in SharePoint. With SharePoint 2013, the search capabilities are heavily invested in and we see a lot of new ways to perform our searches. In this post I’ll show a simple example of how you can utilize the REST Search API’s in SharePoint 2013 to perform a search.
REST Search in SharePoint 2013
So in order to get started, we’ll need to have an ASPX Page containing some simple markup and a javascript file where we’ll put our functions to be executed. The approach mentioned in this post is also compatible with SharePoint Apps, should you decide to develop an App that relys on Search. In my example I’ve created a custom Page which loads my jQuery and JavaScript files, and I’m deploying those files using a Module to the SiteAssets library.
Preview of the simple solution
[
Creating a Search Application using REST Search Api’s
Let’s examine how you can construct your search queries using REST formatting and by simply changing the URL to take in the proper query strings.
Formatting the Url
By formatting the Url properly you can retrieve search results pretty easily using REST.
Query with querytext
If you simply want to return all results with no limits or filters from a search, shoot out this formatted url:
[blockquote]http://tozit.dev/_api/search/query?querytext=’Awesome'[/blockquote]
will yield the following result:
[![image_thumb[1]](/coet/content/images/2012/12/image_thumb11.png)
Essentially we’re getting a bunch of XML returned from the query which we then have to parse and handle somehow. We can then use the result of this query in our application in whatever fashion we want. Let’s see what a very simple application could look like, utilizing the SharePoint 2013 rest search api’s.
ASP.NET Markup Snippet
As we can see in the following code snippet, we’re simply loading a few jQuery and JavaScript files that we require and we define a Search Box and a Button for controlling our little Search application.
JavaScript logic (RestSearch.js)
I’ve added a file to the project called RestSearch.js, which is a custom javascript file containing the following code which will perform an ajax request to SharePoint using the Search API’s:
// in reality we should put this inside our own namespace, but this is just a sample.
var context;
var web;
var user;
// Called from the ASPX Page
function executeSearch()
{
var query = $("#searchBox").val();
// run
SPSearchResults =
{
element: '',
url: '',
init: function(element)
{
SPSearchResults.element = element;
SPSearchResults.url = _spPageContextInfo.webAbsoluteUrl + "/_api/search/query?querytext='" + query + "'";
},
load: function()
{
$.ajax(
{
url: SPSearchResults.url,
method: "GET",
headers:
{
"accept": "application/json;odata=verbose",
},
success: SPSearchResults.onSuccess,
error: SPSearchResults.onError
}
);
},
onSuccess: function (data)
{
var results = data.d.query.PrimaryQueryResult.RelevantResults.Table.Rows.results;
var html = "<div class='results'>";
for (var i = 0; i < results.length; i++)
{
var d = new Date(results[i].Cells.results[8].Value);
var currentDate = d.getFullYear() + "-" + d.getMonth() + "-" + d.getDate() + " " + d.getHours() + ":" + d.getMinutes();
html += "<div class='result-row' style='padding-bottom:5px; border-bottom: 1px solid #c0c0c0;'>";
var clickableLink = "<a href='" + results[i].Cells.results[6].Value + "'>" + results[i].Cells.results[3].Value + "</a><br/><span>Type: " + results[i].Cells.results[17].Value + "</span><br/><span>Modified: " + currentDate + "</span>";
html += clickableLink;
html += "</div>";
}
html += "</div>";
$("#searchResults").html(html);
},
onError: function (err) {
$("#searchResults").html("<h3>An error occured</h3><br/>" + JSON.stringify(err));
}
};
// Call our Init-function
SPSearchResults.init($('#searchResults'));
// Call our Load-function which will post the actual query
SPSearchResults.load();
}
The aforementioned script shows some simple javascript that will call the Search REST API (the "_api/…" part of the query) and then return the results in our html markup. Simple as that.
Summary
By utilizing the REST Search API we can very quickly and easily create an application that searches in SharePoint 2013.
This can be implemened in SharePoint Apps, Sandboxed Solutions or Farm Solutions. Whatever is your preference and requirements, the Search API’s should be easy enough to play around with.
Enjoy.
Recent comments