Web Part Caching – A simple approach
Today we’ve been looking at Web Parts, creation of custom webparts and best practices for creating our custom solutions based on web parts.
I quickly coded up a sample which is caching items in a webpart – Huge server load is a major impact point for some organizations, making caching a strategically important choice if there’s much redundancy.
Anyway, you probably already know that it’s a good thing to cache your data once in a while if you’ve got heavy load and the data isn’t any “to-the-minute” critical information. So let’s get on with it..
Why?
Since people have been asking for a ‘simple sample’ of how to cache things in SharePoint – I thought that I would provide just that, a simple sample.
Check it out!
Web Part fetching items from a SPWeb object, looping all SPList objects and displaying the ItemCount property.
If there isn’t a cache object present, the iteration of the lists will be done immediately
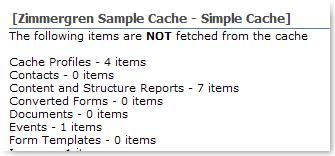
If there is a cache object present, it will fetch the information from the cached object instead of iterating the lists, saving us some resources
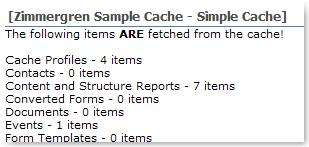
Code It!
This is the full code of the simple sample cache Web Part:
using System;
using System.Collections.Generic;
using System.Text;
using System.Web.UI;
using Microsoft.SharePoint;
using System.Web;
namespace Zimmergren.WebParts.SampleCachePart
{
public class SimpleCache : System.Web.UI.WebControls.WebParts.WebPart
{
protected override void Render(System.Web.UI.HtmlTextWriter writer)
{
base.Render(writer);
List lists = new List();
string status = "";
if (HttpRuntime.Cache["SimpleSampleCache"] == null)
{
status = "The following items are **NOT** fetched from the cache";
SPWeb web = SPContext.Current.Web;
foreach (SPList list in web.Lists)
lists.Add(list);
HttpRuntime.Cache.Add("SimpleSampleCache",
lists,
null,
DateTime.MaxValue,
TimeSpan.FromMinutes(10),
System.Web.Caching.CacheItemPriority.Default, null);
}
else
{
status = "The following items **ARE** fetched from the cache!";
lists = (List)HttpRuntime.Cache["SimpleSampleCache"];
}
writer.Write(status);
foreach (SPList l in lists)
writer.WriteLine(l.Title + " – " + l.ItemCount + " items");
}
}
}
Summary & Download!
I always use the HttpRuntime
or HttpContext
objects to store and read my cached objects. You can of course use the built-in caching functionality of WSS 3.0 Web Parts if you feel that you have the need for it. However this approach works everytime, everywhere. Not just for Web Parts of course, but for any kind of ASP.NET hooked application.
Thanks for tuning in,
Cheers
Recent comments