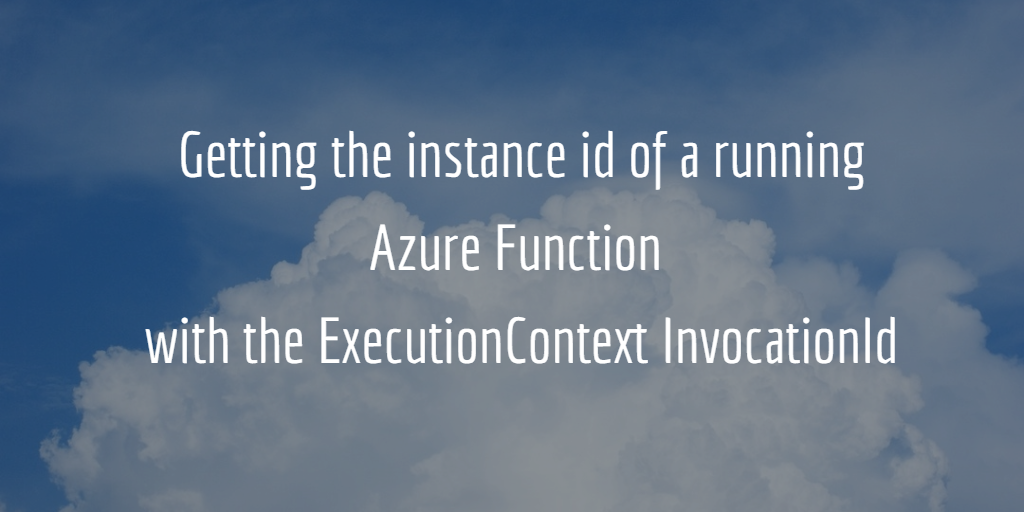
Getting the instance id of a running Azure Function with ExecutionContext.InvocationId
Recently I had a discussion about Azure Functions, the pros and cons, and running multiple instances of batch processing simultaneously.
One of the immediate questions that came up in that discussion was; In the built-in logs you see in the streaming log service or in the log console, how do you know which message comes from what invocation?
Great question, let's dig into that!
Get the Invocation Id of an instance of a Azure Function
When a Function runs, it gets a new unique identifier by default. You can see this in the invocation log:
2016-12-01T22:34:34.605 Function started (Id=6844c578-b2e5-4449-bdde-cee0273106ae)
...
...
...
2016-12-01T22:34:34.699 Function completed (Success, Id=6844c578-b2e5-4449-bdde-cee0273106ae)
There's a guid there, which is the ID of the current instance of the function. But what if you want to use that Id in all your log messages or anywhere else you need it?
Introducing the ExecutionContext
While digging around in the Azure WebJobs SDK, I found the ExecutionContext
object:
GitHub: Azure WebJobs SDK Extensions - ExecutionContext.cs
This file is very simple and has a single job. Getting the invocation id.
In your Azure Function, you can add this to your parameters like this:
public static void Run(string input, ExecutionContext exCtx, TraceWriter log)
{
... your logic here...
}
Now, you can use exCtx.InvocationId
from wherever you want in this method, and you'll get that unique identifier.
Here's a quick example of this in action, to distinguish multiple running functions' log messges in a stream of logs.
using System;
private static string _invocationId;
private static TraceWriter _logger;
public static void Run(string input, ExecutionContext exCtx, TraceWriter log)
{
// Assign the ExecutionContext to a variable we can access later
_invocationId = exCtx.InvocationId.ToString();
// Assign the Log to a variable we can access later
_logger = log;
Log("Hello World");
Log("This is a sample");
Log("I hope it works!");
}
// Using this Log method instead of the log.Info message, appends the invocation id every time. (for demo purposes..)
private static void Log(string message){
_logger.Info($"Function-({_invocationId}): {message}");
}
Should you now run multiple instances of this function, you could distinguish the log messages from a big chunk of data and filter it easily. But that's just a demo use-case; If you need the Function invocation id for something, that's how you get it.
Cheers,
Tobias.
Recent comments