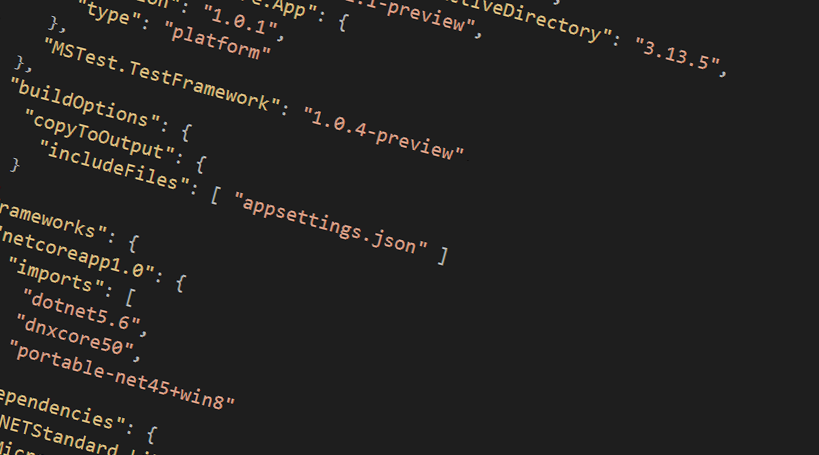
Using appsettings.json instead of web.config in .NET Core projects
Recently I was in a discussion with an acquaintance about transforming their projects into .NET Core from their full .NET applications. Some of these apps have a few core helpers, including the very common requirement to read from config files. Most notably it's the web.config
or app.config
. In this post, I'm simply outlining the minimal steps required to get a grasp on how you can replace those files with the ConfigurationBuilder
in .NET Core, and what your new json
-based configuration files can look like.
Another tip-of-the-day. Enjoy.
Add settings in a new appsettings.json file
Now, one thing that is important is to specify in your project.json
file that the new appsettings.json
file should also be copied to the output folder. Remember how you used to do back in the day, when you selected a file and chose "Copy always" etc? Well, now you specify these things in the json config, which makes it very simple and with a great overview of what actually happens when you build.
Add the following section to your project.json
, of course with the name of your own file(s) you want to include:
"buildOptions": {
"copyToOutput": {
"includeFiles": [ "appsettings.json" ]
}
}
In my example above, I'm simply copying the appsettings.json
file from my project root to the output dir when I compile my project. This enables me to easily find it when running my project.
Contents of appsettings.json
To make it a bit more clear, here's the full content of my very simple appsettings.json
file:
{
"StorageConnectionString": "UseDevelopmentStorage=true",
"TestSetting1": "Tobi 1 Kenobi",
"TestSetting2": {
"SubSetting1": "Hello",
"SubSetting2": "World"
}
}
I've added an example of a simple nested configuration here to demonstrate how that works.
Get your config values using ConfigurationBuilder
If you're in a normal class library or Console App, and you don't have the fancy Startup()
method everyone is talking about, never fear - the code is here. You don't have to put the config inside of the Startup method to access these configurations; Simply use the ConfigurationBuilder
from wherever you want.
Here's what I'm doing in one of my class libraries, which in turn is ran from a console app:
public static IConfiguration GetConfig()
{
var builder = new ConfigurationBuilder()
.SetBasePath(System.AppContext.BaseDirectory)
.AddJsonFile("appsettings.json",
optional: true,
reloadOnChange: true);
return builder.Build();
}
Let's break that down a bit.
SetBasePath
This is where the ConfigurationManager will look for your files. Since I've already specified in my project.json
that the appsettings.json
file should be copied to the output, I can simply set the base path to the output dir, which brings me to the next one.
System.AppContext.BaseDirectory
This is a way to get the current directory of your executing assembly. If there's other ways to easily get this, which are more up to date with the .NET Core framework, I'd be happy to hear about it in the comments and I would update it. For now, this seems like a valid approach and works in all of my projects.
AddJsonFile
We're going to have to point to our configuration files. In my case I have the appsettings.json
file, and this is where you'll let the ConfigurationBuilder know that you want to point to it.
Get properties from config
Now that we have the IConfiguration
object (returned from the static method above, for example), it's very easy to work with those values:
Getting the values from your config file is straight forward. Not much different from the traditional ConfigurationManager
approach. Simply get the configuration object (as returned from the method I build above in this post) and point to the properties.
Here's a simple test method, making sure that the values come out correctly from this appsettings.json file using the ConfigurationBuilder that we constructed above:
[TestMethod]
public void ConfigurationHelperTests_GetConfigurationFromAppSettingsJson()
{
var settings = ConfigurationHelper.GetConfig();
Assert.IsNotNull(settings);
Assert.AreEqual("UseDevelopmentStorage=true", settings["StorageConnectionString"]);
Assert.AreEqual("Tobi 1 Kenobi", settings["TestSetting1"]);
Assert.AreEqual("Hello", settings["TestSetting2:SubSetting1"]);
Assert.AreEqual("World", settings["TestSetting2:SubSetting2"]);
}
Summary
That's about it for this post. A simple way to check out how your can replace the logic of your old projects that used ConfigurationManager
and instead use the new ConfigurationBuilder
and point to whatever config file you want.
There's plenty of more ways and gotchas that I haven't had time to document; But upon request I'm putting this out here so there's a starting point.
Check out the section below on resources to read more about how you can work with configuration and settings in your .NET Core projects.
Recent comments