Extending the LINQ to SharePoint context to allow additional fields and properties in your queries
One of the culprits of working with the LINQ to SharePoint contexts that are being auto-generated for us is that it actually doesn’t necessarily render all the fields or properties you require. For example the built-in fields "Created By" or the "Attachments" property of a list item. Oftentimes we need these fields in our queries, whether they be CAML queries or LINQ queries. With LINQ to SharePoint you have the ability to extend an Entity that you’ve generated, and allow additional code to be executed for those entities. In this article I’ll talk briefly about how you can create and manage an extension to your LINQ context to allow additional fields in your queries.
One of the powerful advantages of doing this is that you post-deployment can extend your queries. Lets for example say that the list in your deployment gets some additional fields in the future, then perhaps you’ll need to make sure that your LINQ context can cope with querying those fields as well – this can easily be done by extending an entity context as I’ll talk about below.
In this article I’ll give you a sample of how to extend the LINQ entity to fit our custom needs, including mapping the "Created By" and "Modified By" fields and some additional logical customizations.
Generate your context
First and foremost we’ll need to generate our context. This can be done using SPMetal (Which you can read more about in this article: Getting Started with LINQ to SharePoint), or for example the extensions in CKS:Dev.
I’m going to generate a quick and easy sample for this by utilizing the CKS:Dev tools and then build my samples on top of that.
If you haven’t installed the CKS:Dev tools yet, go right ahead and do that – then get back to this step!
- Generate a LINQ context by using the CKS:Dev plugin, which will give you this additional context menu in the SharePoint server browser:
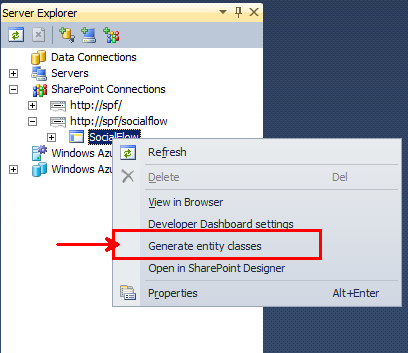
This should auto-generate a class file for you, containing all the entities for the selected site:
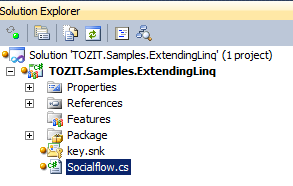
The file contains all the LINQ to SharePoint data context information that a normal SPMetal.exe command would generate, since this tool in essense is using SPMetal.exe:
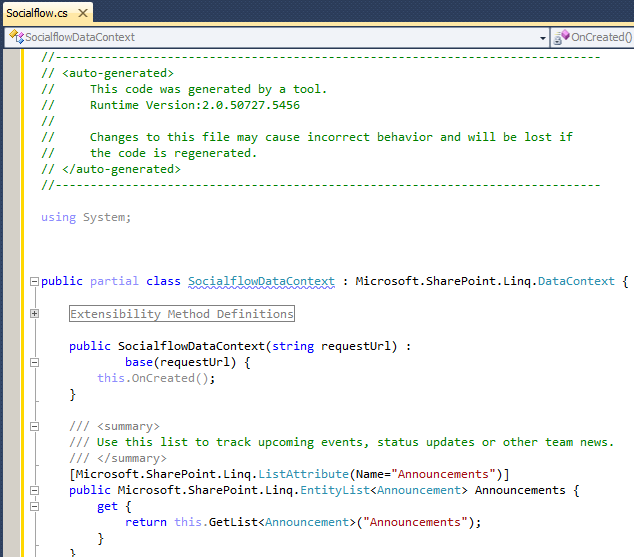
As you can see, we’ve easily created the LINQ data context that we need, but unfortunately it’s missing some vital parts for our application – namely some of the built-in fields that you would normally want to work with. In my case, I was lacking the "Created By" and "Modified By" fields and I’d have to find a way to extend the LINQ context to cope with this so my queries can be easily constructed even for those fields.
Extending an entity in your generated LINQ context
In order for us to be able to extend the LINQ context, Microsoft provides us with an interface called ICustomMapping.
We’ll start by inheriting a new class from this interface, and name the class along the lines of the entity we want to extend.
namespace TOZIT.Samples.ExtendingLinq
{
using Microsoft.SharePoint.Linq;
///
/// A LINQ extension to the Announcement-entity
///
public partial class Announcement : ICustomMapping
{
public void MapFrom(object listItem)
{
throw new System.NotImplementedException();
}
public void MapTo(object listItem)
{
throw new System.NotImplementedException();
}
public void Resolve(RefreshMode mode, object originalListItem, object databaseListItem)
{
throw new System.NotImplementedException();
}
}
}
Make sure you follow these rules:
- Class name should be the same name as the entity you’re extending (partial class)
Let’s go ahead and add the necessary code to extend our entity. I’ll throw in some simple sample code here on how to map the fields we want, and to add additional logic to our new extension.
namespace TOZIT.Samples.ExtendingLinq
{
using Microsoft.SharePoint;
using Microsoft.SharePoint.Linq;
///
/// A LINQ extension to the Announcement-entity
///
public partial class Announcement : ICustomMapping
{
#region Properties
///
/// Gets or sets the Created Byproperty
///
public string CreatedBy { get; set; }
///
/// Gets or sets the Created By Login Name property
/// Returns a reader-friendly version of the user's loginname
///
public string CreatedByLoginName { get; internal set; }
///
/// Gets or sets the Modified By property
///
public string ModifiedBy { get; set; }
///
/// Gets or sets the Modified By Login Name property
/// Returns a reader-friendly version of the user's login name
///
public string ModifiedByLoginName { get; internal set; }
#endregion
#region Methods
///
/// Assigns a field (column) to a property so that LINQ to SharePoint can read data
/// from the field in the content database to the property that represents it.
///
///
[CustomMapping(Columns = new[] { "Editor", "Author" })]
// Needs to be the InternalName of fields..
public void MapFrom(object listItem)
{
var lItem = listItem as SPListItem;
if (lItem != null)
{
// = MAP THE AUTHOR-FIELD =
// Map the Created By field to the Author (Created By) field
CreatedBy = lItem["Author"].ToString();
// Map the CreatedByLoginName field to the Author's actual LoginName
SPField authorField = lItem.Fields.GetFieldByInternalName("Author");
var authorFieldValue = authorField.GetFieldValue(lItem["Author"].ToString()) as SPFieldUserValue;
if (authorFieldValue != null)
{
CreatedByLoginName = authorFieldValue.User.LoginName;
}
// = MAP THE EDITOR-FIELD =
// Map the Modified By field to the Editor (Modified By) field
ModifiedBy = lItem["Editor"].ToString();
// Map the ModifiedByLoginName field to the Editor's actual LoginName
SPField editorField = lItem.Fields.GetFieldByInternalName("Editor");
var editorFieldValue = editorField.GetFieldValue(lItem["Editor"].ToString()) as SPFieldUserValue;
if (editorFieldValue != null)
{
ModifiedByLoginName = editorFieldValue.User.LoginName;
}
}
}
///
/// Assigns a property to a field (column) so that LINQ to SharePoint can save
/// the value of the property to the field in the content database.
///
/// List Item
public void MapTo(object listItem)
{
var lItem = listItem as SPListItem;
if (lItem != null)
{
lItem["Author"] = CreatedBy;
lItem["Editor"] = ModifiedBy;
}
}
///
/// Resolves discrepancies in the values of one or more fields in a list item with respect to its current client value,
/// its current value in the database,
/// and its value when originally retrieved from the database
///
/// Read more: <http://msdn.microsoft.com/en-us/library/microsoft.sharepoint.linq.icustommapping.resolve.aspx>
///
/// Refresh Mode
/// Original list item
/// Database object
public void Resolve(RefreshMode mode, object originalListItem, object databaseObject)
{
var origListItem = (SPListItem)originalListItem;
var dbListItem = (SPListItem)databaseObject;
var originalCreatedByValue = (string)origListItem["Author"];
var dbCreatedByValue = (string)dbListItem["Author"];
var originalModifiedByValue = (string)origListItem["Editor"];
var dbModifiedByValue = (string)dbListItem["Editor"];
if (mode RefreshMode.KeepCurrentValues)
{
// Save the Current values
dbListItem["Author"] = CreatedBy;
dbListItem["Editor"] = ModifiedBy;
}
else if (mode RefreshMode.KeepChanges)
{
// Keep the changes being made
if (CreatedBy != originalCreatedByValue)
dbListItem["Author"] = CreatedBy;
else if (CreatedBy originalCreatedByValue && CreatedBy != dbCreatedByValue)
CreatedBy = dbCreatedByValue;
if (ModifiedBy != originalModifiedByValue)
dbListItem["Editor"] = ModifiedBy;
else if (ModifiedBy originalModifiedByValue && ModifiedBy != dbModifiedByValue)
ModifiedBy = dbModifiedByValue;
}
else if (mode RefreshMode.OverwriteCurrentValues)
{
// Save the Database values
CreatedBy = dbCreatedByValue;
ModifiedBy = dbModifiedByValue;
}
}
#endregion
}
}
As you can see in my sample above, I not only mapped the two fields I need but I also slightly extended the entity with additional properties for retrieving a clean LoginName from the user-objects. Obviously this can be done using a normal .NET 3.5 extension method on the SPUser object, but here I implemented it in the context to show how it can be easily extended to fit our needs.
Writing a query with our extended data context
So if we’ve followed the very simple steps of creating a new extension for one of our entities, we can now easily access these details from the query we’re writing:
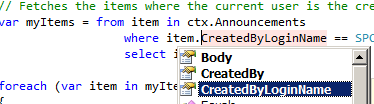
using System.ComponentModel;
using System.Web.UI.WebControls.WebParts;
using Microsoft.SharePoint;
namespace TOZIT.Samples.ExtendingLinq.LinqWebPart
{
using System.Linq;
using System.Web.UI.WebControls;
[ToolboxItemAttribute(false)]
public class LinqWebPart : WebPart
{
protected override void CreateChildControls()
{
using (var ctx = new SocialflowDataContext(SPContext.Current.Web.Url))
{
// Fetches the items where the current user is the creator
var myItems = from item in ctx.Announcements
where item.CreatedByLoginName SPContext.Current.Web.CurrentUser.LoginName
select item;
foreach (var item in myItems)
{
Controls.Add(new Literal { Text = item.Title + " : " + item.CreatedByLoginName + " (you) created this item" });
}
}
}
}
}
The list contains a few items (all created by the current user, which is the system account – please use your imagination… :-)
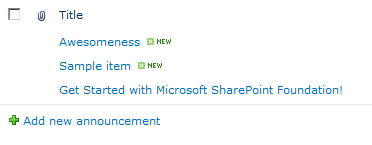
And the results:

Summary
All in all, this gives us the flexibility to customize the way we do our queries in SharePoint using LINQ. I’ve gotten the question about extending LINQ to SharePoint quite a few times over the past years, so I thought it’d be time to reflect those thoughts in this post. I hope you enjoy it and can start utilizing the extension of your LINQ queries!
Enjoy!
Recent comments