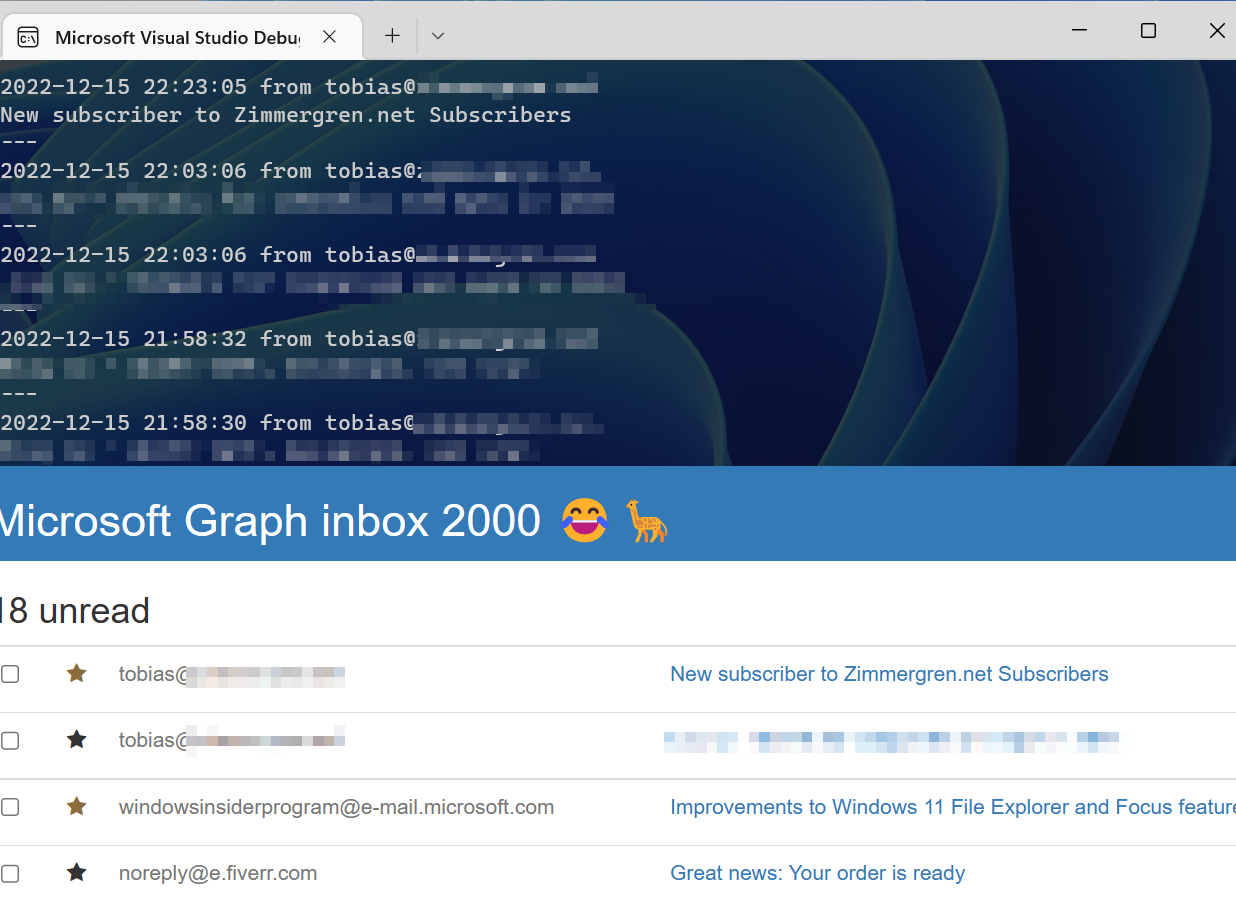
Reading e-mails with Microsoft Graph using .NET
Table of Contents
Following a popular article I published on Sending e-mails with Microsoft Graph using .NET, I have been asked on several occasions how to read e-mails from an inbox.
There are several ways to accomplish this, and I'm highlighting one of the easier ways to show a working code example. You can twist and turn it to your liking to fit your solutions.
The setup
I'm outlining some prerequisites for you to set up, including a new Azure AD application and the required permissions.
As a general prerequisite, reading the "Setup" section of my previous article is helpful.
First, create an Azure AD application
I've written a guide for how to accomplish this already. Read the following section of a previous article:
Secondly, modify the permissions of the new app
In the previous link, you saw how to set the permissions of your new app. Instead of using Mail.Send
, we need to set it to Mail.Read
.
I'm using Application Permissions for this demo, as opposed to delegated, which means my application can read any user's inbox.
- Remember the shared responsibility model for security – it's in you to protect your secrets, applications, and users' data!
- ⚠️ Be careful and restrictive with application permissions, as they can be very permissive and grant access to a lot of data.
The code
The code is available on GitHub.
Install dependencies (NuGet)
These are the only NuGet packages you need:
For development: Secrets.json
As with the previous article, I'm storing my dev secrets in the secrets.json file for this demo.
{
"GraphMail": {
"TenantId": "",
"ClientId": "",
"ClientSecret": ""
}
}
If you opt to use user secrets during development, you need to insert the values from your Azure AD application in this section. I still recommend using a managed identity to avoid credentials in code, config, and anything that flies in production.
The C# code
Contrary to the previous article I published on the topic; this is a lighter example; therefore, the code is straightforward.
using Azure.Identity;
using Microsoft.Extensions.Configuration;
using Microsoft.Graph;
namespace TZ.GraphReadEmailDemo
{
class Program
{
// A simple demonstration of how to read e-mails from an Inbox using the Microsoft Graph
// The code example uses the Mail.Read permission to access the current user's inbox.
// Read the blog post: https://zimmergren.net/reading-emails-with-microsoft-graph-using-net/
static void Main(string[] args)
{
/*
* IMPORTANT:
* This is demo code only.
* Authentication should preferably be done using managed identity, without any credentials in code or config.
* */
// Set up the config to load the user secrets
var config = new ConfigurationBuilder()
.SetBasePath(AppDomain.CurrentDomain.BaseDirectory)
.AddUserSecrets<Program>()
.Build();
// Define the credentials.
// Note: In your implementations of this code, please consider using managed identities, and avoid credentials in code or config.
var credentials = new ClientSecretCredential(
config["GraphMail:TenantId"],
config["GraphMail:ClientId"],
config["GraphMail:ClientSecret"],
new TokenCredentialOptions { AuthorityHost = AzureAuthorityHosts.AzurePublicCloud });
// Define a new Microsoft Graph service client.
GraphServiceClient _graphServiceClient = new GraphServiceClient(credentials);
// Get the e-mails for a specific user.
var messages = _graphServiceClient.Users["user@e-mail.com"].Messages.Request().GetAsync().Result;
foreach (var message in messages)
{
Console.WriteLine($"{message.ReceivedDateTime?.ToString("yyyy-MM-dd HH:mm:ss")} from {message.From.EmailAddress.Address}");
Console.WriteLine($"{message.Subject}");
Console.WriteLine("---");
}
}
}
}
The results
I can't claim I'm a great UI designer, but the data is available, and you can now do with it what you need.
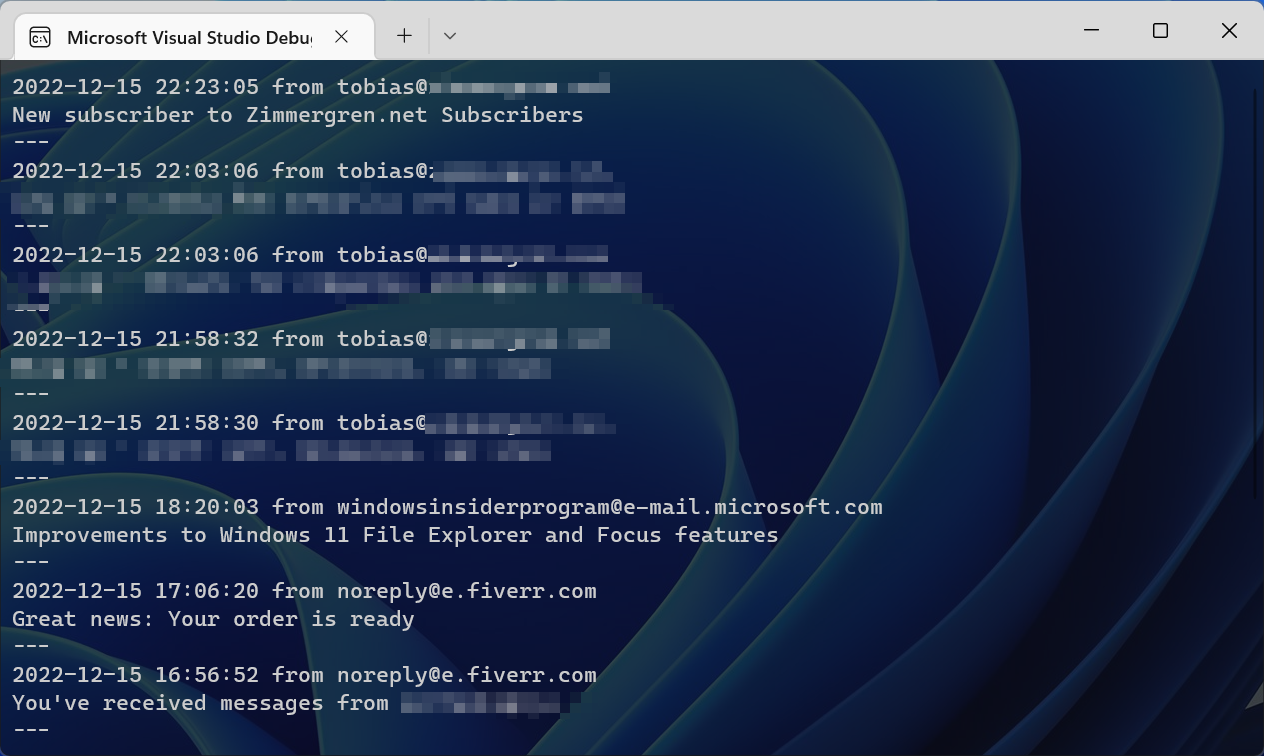
Make it (more) pretty
While I've already claimed that I'm no UI designer, the very least I can do is plug the code into a working HTML template to showcase a slightly more beneficial scenario.
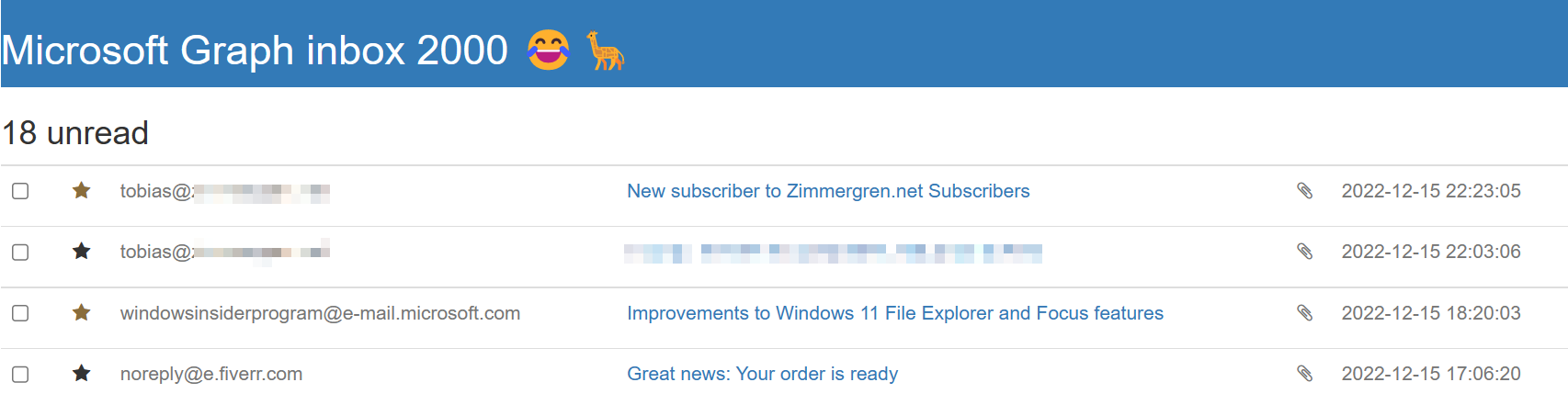
I'm glad I don't work with front-end development, but it's fun to showcase a great SDK that makes things easy for you.
Summary
Easy as 1-2-3. An application that reads e-mails from Microsoft Graph using .NET is easy to craft. The fun challenges ahead are the use cases and putting this into practice, and designing for scale and security.
More relevant content for Microsoft Graph:
Check out the Azure Well-Architected Framework and the five pillars for building awesome applications running on Azure:
Recent comments